Introduction:
In the world of Angular development, managing application state efficiently is crucial for building scalable and maintainable applications. As applications grow in complexity, managing the state becomes increasingly challenging. This is where the NgRx Store comes into play. NgRx Store is a powerful library for managing state in Angular applications, based on the Redux pattern.
What is NgRx Store?
At its core, NgRx Store follows the principles of the Redux pattern, which emphasizes a single source of truth, immutable state, and unidirectional data flow. Let's break down these principles:
• Single Source of Truth: NgRx Store maintains a single source of truth for the application state. This centralization simplifies state management and ensures consistency across the application.
• Immutable State: In NgRx Store, the state is immutable, meaning it cannot be directly modified. Instead, state changes are achieved by dispatching actions, which are handled by reducers to produce a new immutable state.
• Unidirectional Data Flow: NgRx Store enforces a unidirectional data flow, where state changes flow in one direction—from actions dispatched by components to reducers, which update the state, and finally to components that subscribe to the updated state.
Core Concepts:
Actions: Actions are payloads of information that describe an event or user interaction within the application. They are dispatched by components to trigger state changes. Actions are defined using simple
classes or functions and are typically represented as plain objects with a type property.
- Reducers: Reducers are pure functions responsible for handling state transitions based on dispatched actions. A reducer takes the current state and an action as arguments and returns a new state. Reducers should be pure functions with no side effects, ensuring predictability and testability.
- Selectors: Selectors are pure functions used for obtaining specific slices of state from the store. They provide a way to encapsulate access to the store state and derive computed values or derived state. Selectors improve performance by memoizing results and optimizing re-rendering.
- Effects: Effects are used to handle side effects, such as asynchronous operations and external API calls. Effects listen for dispatched actions and perform tasks accordingly.
Usage in Angular Applications:
Setup NgRx Store in app.module.ts:
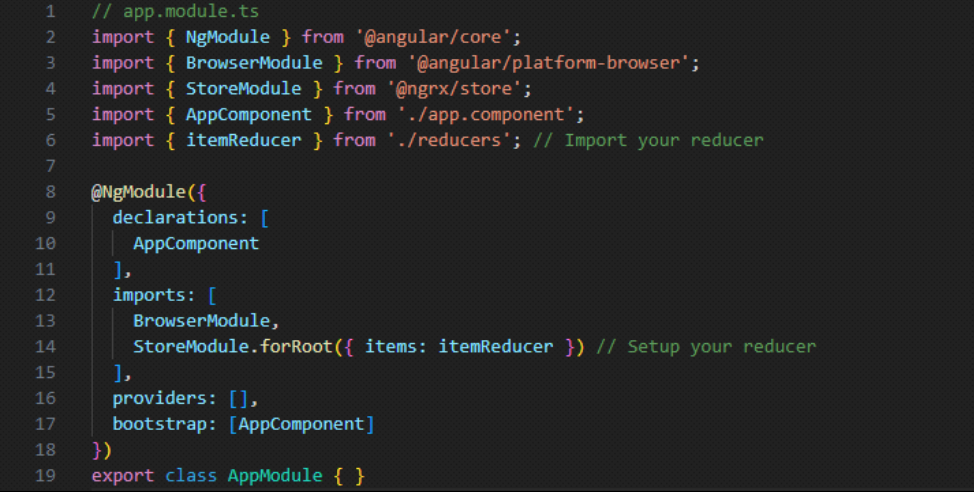
Creating an Action and Reducer:
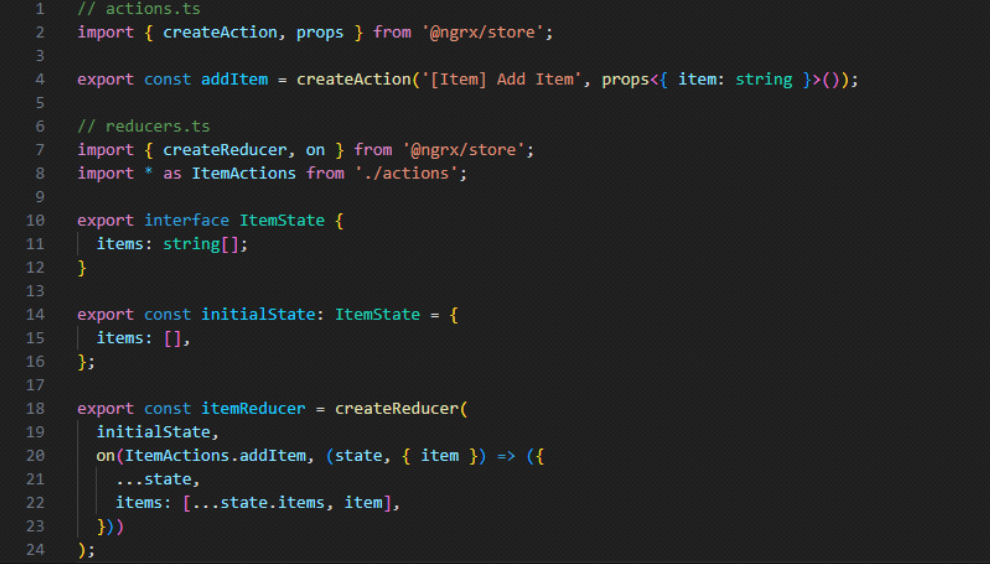
Integration with components:
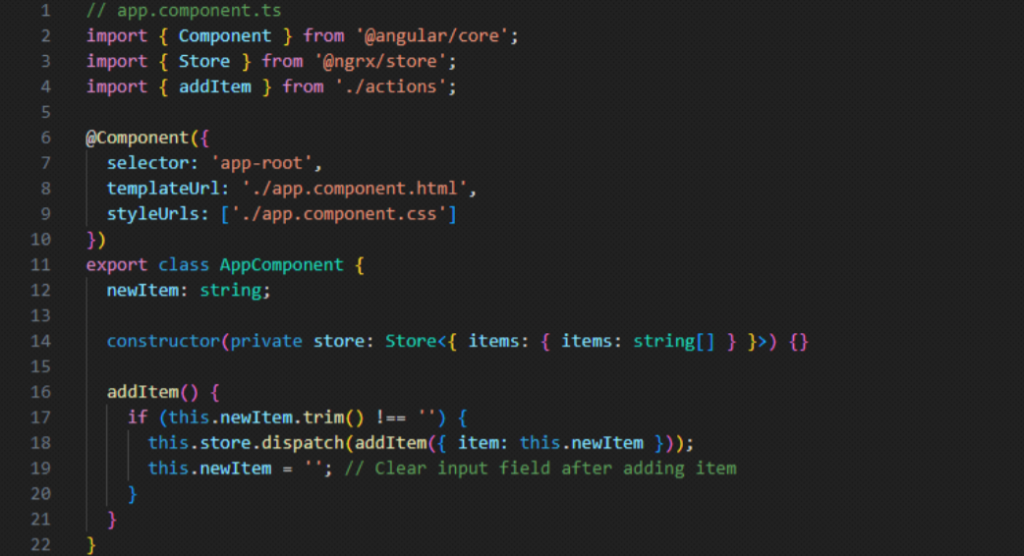
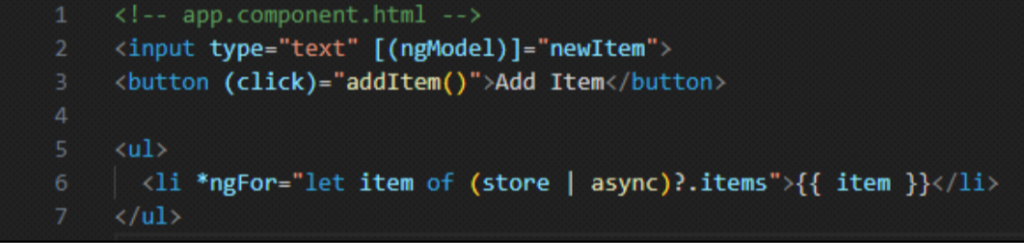
Benefits of NgRx Store:
NgRx Store offers several benefits for managing state in Angular applications:
- Centralized State Management: NgRx Store centralizes state management, making it easier to understand and maintain the application state.
- Predictable State Changes: The Redux pattern ensures predictable state changes, making it easier to debug and reason about application behavior.
- Improved Debugging and Testing: NgRx Store enhances debugging and testing by providing clear and traceable state changes. It promotes testability by isolating side effects and application logic.
Conclusion:
NgRx Store is a powerful library for managing state in Angular applications, based on the Redux pattern. By following the core principles of a single source of truth, immutable state, and unidirectional data flow, NgRx Store simplifies state management and promotes predictable application behavior. With the benefits of centralized state management, predictable state changes, and improved debugging and testing, NgRx Store is a valuable tool for building scalable and maintainable Angular applications.