Overview:
Considering the developers’ angle of choosing the right software library and framework that are easy and fun to use are preferred. Among the React community, Next Js has become a popular choice for developers who want to get started quickly. Next Js builds on top of React to provide a streamlined development experience, although there is a slight learning curve.
This blog talks about both React Js and Next Js, the comparison between both, advantages, features, etc.
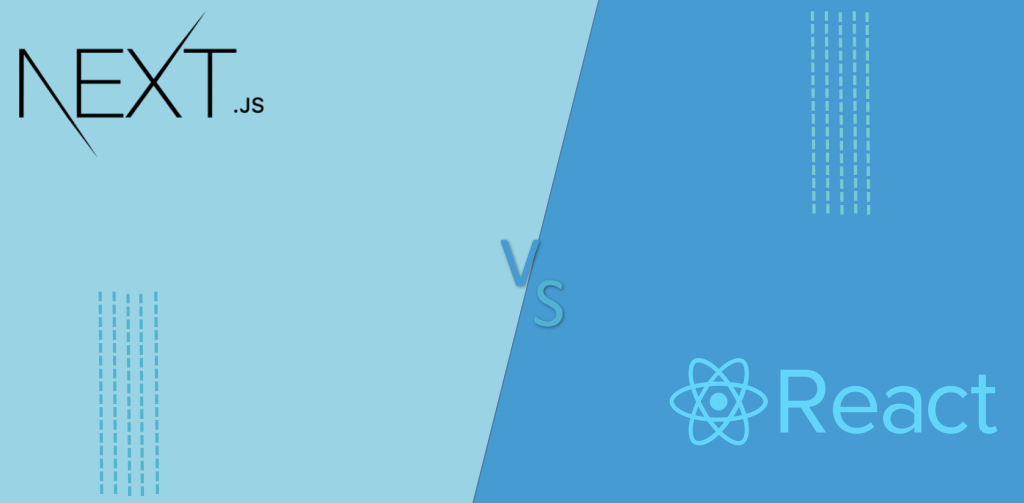
What is Next Js?
Next Js is an open-source JavaScript framework which is created by Vercel. Right out of the box, Next Js provides things like pre-rendering, routing, code splitting, and webpack support. One of Next Js’s key features is its ability to automatically code-split your application, meaning that each page only loads the necessary JavaScript for that page view. This results in faster page loads and an improved user experience.
Advantages:
- Speed- Next Js supports static site generation and server-side rendering. Static generation is fast because all web application pages have been pre-rendered, cached, and served over a CDN.
- Less setup- In Next Js, most features you get come with zero configuration as they are inbuilt. For example, page routing is where you do not need to write any code to create a route in your app.
- Easily create your own backend – Easily create your custom back-end functionalities
to power your own front-end. This does not affect the size bundle of your client-side application. - Built-in CSS support – One of the key features of Next Js is its built-in CSS support. This means that developers can include CSS Stylesheets within their Next Js projects without needing to use any additional libraries or tooling.
What is React Js?
React was originally created by Facebook( Meta) and has become one of the most popular libraries in the frontend world today. React is easily extendable and can include features like routing as well as state management patterns with libraries like Redux. React components can be stateless or stateful and only re-render within the scope of the applied state as the entire page does not reload when something is clicked. In the traditional approach, the whole page reloads to show another state which in most cases can be slow to reload. The concept of using React components stops the reprocessing of every code or logic for that particular page.
Advantages:
- Easy to learn- Currently, the educational state of React Js is good because, over the years, React has grown with its community, and the community has made thousands of materials available for reference. The good supply of documentation and tutorial videos make React Js a good catch.
- Javascript Syntax extension- JSX is a JavaScript syntax extension that makes writing dynamic web apps in React Js easier. JSX code is compiled into JavaScript, and the Babel compiler will automatically optimize your code for performance.
- Reusable Components- React is a powerful JavaScript library that enables developers to create Reusable User Interfaces (UI). A key feature of React is the ability to create Components, which are self-contained units of code that can be reused throughout your app.
- Performance- One of the reasons for its success is its performance. React uses a virtual DOM to manage updates to the user interface. This makes React apps fast and responsive. When a user interacts with a React app, only the relevant parts of the DOM are updated. This means that there is no need to redraw the entire page. This makes React apps much faster than traditional JavaScript frameworks.
- Seo Friendly- React Js is a JavaScript library that is used for building user interfaces. It is also known for its speedy performance and small size. React Js is SEO-friendly because it uses server-side rendering. This means that the content of your React JS application will be rendered on the server before it is sent to the client.
Difference between Next js and React Js:
Next is a framework for React which is built upon the React library.
React is a library, not a framework.
Next is famous for Server-side rendering and static generation of websites.
React on the other side doesn’t support Server-side rendering.
Next can be difficult for someone to learn without prior React Js knowledge.
React can be easier to learn as compared to Next Js.
The web apps built using Next Js are very fast.
The web apps built using React Js are slow as compared to Next Js.
With Next Js, we can build an entire web application.
React Js helps in building the beautiful UI of a web application.
In the Next Js public folder there is no index.html file as an HTML file will be made in Next Js according to the type of need.
In React Js single HTML file index.html is present in a public folder that manages the whole React app.
Client-side Rendering (CSR) in React:
import React from 'react&';
const Counter = () => {
const [count, setCount] = React.useState(0);
const incrementCount = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}<p>
<button onClick={incrementCount}>Increment</button>
</div>
);
};
export default Counter;
As seen above, the Counter component uses React’s useState hook to manage the count state. The count is updated in the client’s browser, and the UI is re-rendered accordingly whenever the user clicks the “increment” button.
Server-side Rendering (SSR) in Next.js:
import React from 'react';
const Counter = () => {
const [count, setCount] = React.useState(0);
const incrementCount = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={incrementCount}>Increment</button>
</div>
);
};
export default Counter;
In Next Js’s code, the Counter component is the same as the React example. But, with Next. Jsm, this component can be rendered on the server and sent to the client as pre-rendered HTML.
Features of Next Js:
The following features make Nextjs a cutting-edge tool for developers:
File system routing- The essential feature of Next Js that enables developers to route files more easily and effectively. Next Js allows the mapping of URLs directly to the files in the project’s directory. This enables files to become routes and streamline navigation more swiftly.
Server-Side Rendering- Next Js supports the rendering of pages on user requests on the server-side by generating a non-interactive HTML, while React uses JSON data and JavaScript instruction to make the page interactive on the client-side.
Static site generator- Statically generated web pages are said to be SEO optimized because of their speed, which can make Google rank that page higher. With Next Js supporting static page generation, it stands out against React.
Image optimization- The HTML <img> tag has been evolved by the Next Js team with built-in performance to help with Picture optimization. To use this feature, the next/image component is imported. With this feature, images automatically resize to the screen size seamlessly, even images from a remote location. This new feature provides developers with an easy way to optimize their images for performance without having to manually resize or compress them.
Automatic code splitting- As your Next Js applications grow bigger, the size of a third-party library, CSS, and JavaScript files or bundles increases. Instead of downloading a large file on page load, these code/scripts can be split into smaller units, and for every feature required, these scripts are downloaded immediately, thereby increasing performance.
Type script support- Typescript is a superset of JavaScript that adds type checking and other features that can help to improve the quality of code. While Typescript is not required for Next Js, it can be a valuable tool for developers looking to improve the quality of their code.
API route- Next Js provides a built-in way to create your APIs, called API routes. With API routes, you can create your own endpoints and handle incoming requests however you want. You can use API routes to create a custom backend for your Next Js application or to expose data from your database to the front end. Either way, API routes give you a lot of flexibility in how you build your Next Js application.
Features of React Js:
The following features made React edge over other frameworks:
JavaScript Syntax Extension (JSX)- JSX is a combination of JavaScript and HTML. This syntax extension is basically used to create React elements.
Component- Everything in React is a component. Multiple React components are coupled together to create simple user interfaces for very large and complex UI. Each of these components can have its logic and behaviors. Components are reusable in any part of the web page by just calling it.
Virtual DOM- This is one of the best features of React Js, which speeds up the app development process while still providing flexibility. DOM stands for Document Object Model. The approach allows a web page to be replicated in React’s virtual memory. A virtual DOM object represents the original DOM object.
One-way data binding- React Js follows unidirectional data flow, also known as one-way data binding. React uses a unidirectional data flow, forcing developers to use the callback function to edit components rather than directly altering them. Flux is a Js app design component that allows you to control data flow from a single point.
Simplicity- React Js uses a JSX file, which makes the application easy to code and comprehend. React Js is a component-based approach, which means the code may be reused as needed. React Js makes use of JSX, a hybrid of HTML and JavaScript. This reduces the amount of code and makes it easier to understand and debug.
Performance- React Js is well-known for its speed. These characteristics sets React apart from the other frameworks that are available today. This is because it controls a virtual DOM. The Document Object Model (DOM) is a computer programming API for HTML, XML, and XHTML that is cross-platform. The DOM exists solely in memory.
Conclusion:
What do we choose, React Js or Next Js?
Well, you will not find any accurate answer to this question. Having said earlier, selecting a framework or library completely depends on your project and developer. React and Next Js are emerging and beneficial tools for your project, but only for performing certain tasks.
When you go with Next Js, it offers the best server-side rendering and static website development solutions. Also, it lets you manage projects easily with various tools and features.
On the other hand, React is the best choice to develop user interfaces for single-page applications. Since it works with the layer of mobile and web apps, it allows you to create more appealing and intuitive applications.
In a nutshell, Next Js offers various tools and features to minimize the development process, whereas, React Js has better resources for front-end development of your mobile and web application.